This is the fourth in a series of articles about using microcontroller devices with e-paper displays for displaying dynamic calendars.
The custom e-Paper Featherwing I created has been working great with the Waveshare family of e-Paper modules. As a fan of Adafruit products, I was curious if it was possible to get it to also work with Adafruit’s line of e-Paper modules. Adafruit ( https://Adafruit.com ) has several tri-color (black, white and red) e-Paper devices ranging from 1.54″ to 2.7″ diagonal screens. Happily, it works! So the same custom Paper Featherwing can now work with either Waveshare or Adafruit e-Paper displays. This article will detail how to use the Featherwing with the Adafruit displays. Note: The custom e-Paper Featherwing is available at my Tindie store.
First, there are a few caveats using the custom Featherwing with an Adafruit e-Paper display. Since the custom Featherwing supports 8 wires and the e-Paper display has 13 pins, there are not enough pins available to use with the Adafruit display. So, some trade-offs were made. One trade off is the built-in memory on the Adafruit display board is not used. All screen memory must be supplied by the microcontroller. This may be an issue if memory is at a premium on your microcontroller. Also, there is no pin available for reading the built-in SD Card slot on the display. On the plus side, howerver, there are 3 buttons available for use on the Featherwing, a reset button and two programmable buttons.
As a pleasant surprise, the wires connecting the Featherwing to the Adafruit display nearly matched the order of the Waveshare display. Only one wire needed to be crossed. To hook up the Adafruit display, I soldered 13 right angle header pins to the display and used 3″ female/female jumper wires to connect to the display. Straight header pins could also be used.
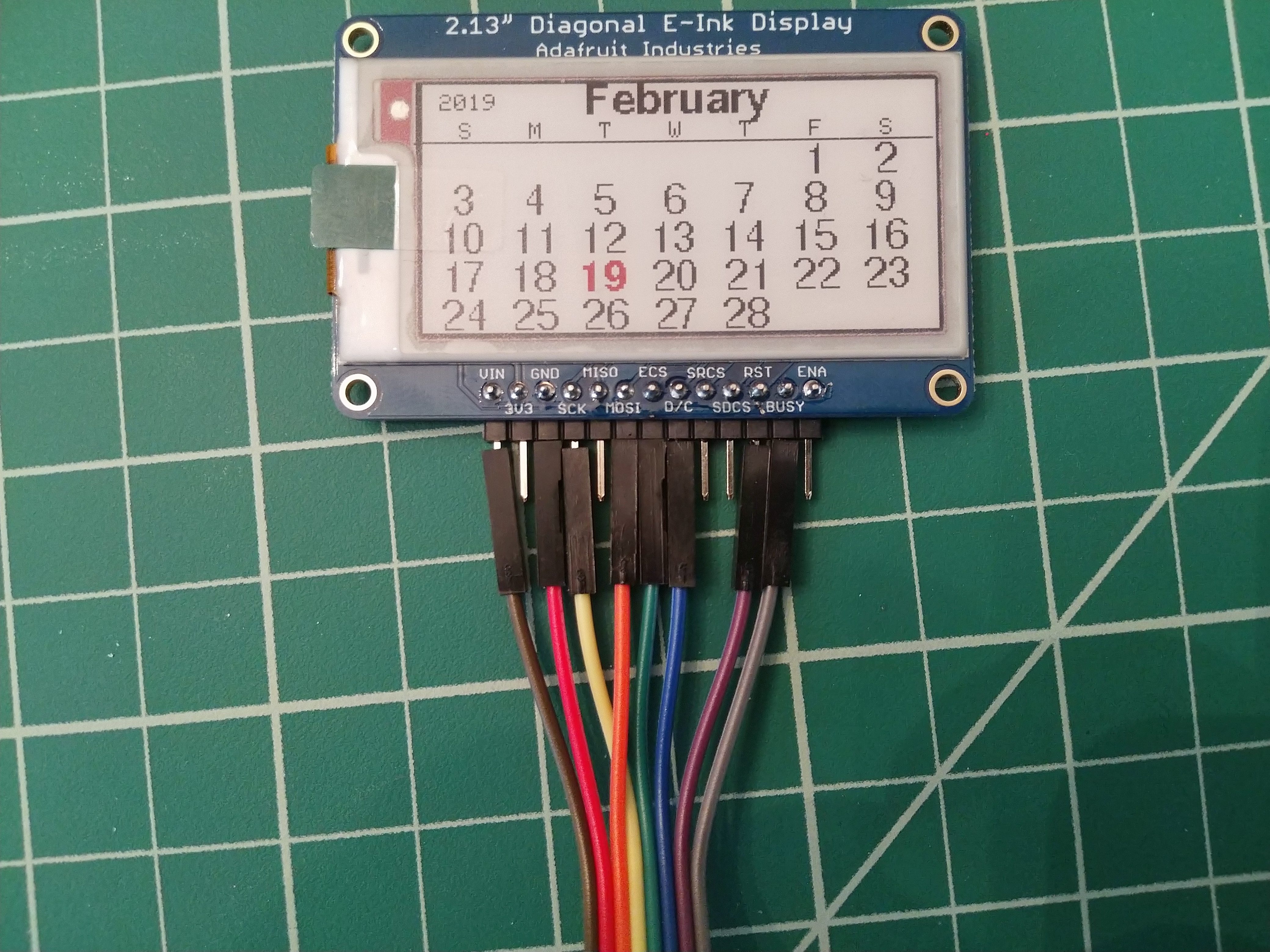
All Adafruit displays use the same pin layout. The pins used on the device are shown in Table 1.
Adafruit Display | Featherwing Pin (Pin Order) |
VIN | VCC (1) |
GND | GND (2) |
SCK | CLK (4) |
MOSI | DIN (3) |
ECS | CS (5) |
D/C | DC (6) |
RST | RST (7) |
BUSY | BUSY (8) |
Table 1: Pin mapping from Adafruit display to Featherwing (note pins 3 and 4 are swapped).
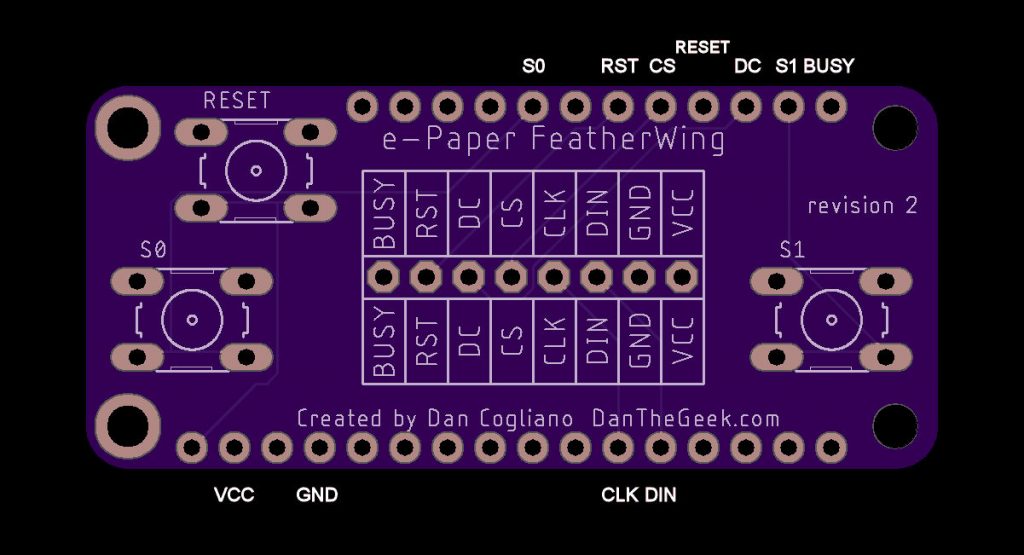
Once I had the I/O pins mapped, I needed to work on getting the e-paper demo program in the Adafruit e-paper library working. This page details how to install the two libraries needed for the display. For this project, I had to make some changes to get it to work with the Featherwing. First, I replaced the default pins in the demo program with the newly mapped ones. I also included the I/O pins for the buttons and LED. They were not needed for this sketch, but they can be used in other sketches using the display.
#if defined(ESP8266)
// Feather HUZZAH 8266
#define EPD_RESET 15
#define EPD_DC 2
#define EPD_CS 0
#define EPD_BUSY 4
#define BUTTON0 12
#define BUTTON1 5
#define LEDPIN 0
#define LEDPINON LOW
#define LEDPINOFF HIGH
#else
// Feather HUZZAH 32 (same physical locations as 8266 but different IO pins)
#define EPD_RESET 33
#define EPD_DC 14
#define EPD_CS 15
#define EPD_BUSY 23
#define BUTTON0 12
#define BUTTON1 22
#define LEDPIN 13
#define LEDPINON HIGH
#define LEDPINOFF LOW
#endif
I also needed to switch the ePaper class constructors to use the ones not using the external SRAM feature. I used these classes instead (uncomment the appropriate one for your ePaper display):
/* Uncomment the following line if you are using 1.54" tricolor EPD */
//Adafruit_IL0373 display(152, 152 ,EPD_DC, EPD_RESET, EPD_CS, EPD_BUSY);
/* Uncomment the following line if you are using 2.15" tricolor EPD */
Adafruit_IL0373 display(212, 104 ,EPD_DC, EPD_RESET, EPD_CS, EPD_BUSY);
/* Uncomment the following line if you are using 2.7" tricolor EPD */
//Adafruit_IL91874 display(264, 176 ,EPD_DC, EPD_RESET, EPD_CS, EPD_BUSY);
Finally, I had to modify the library to not use the external SRAM feature built into the display. I did not see a way to disable it other than modifying the library code itself. This required commenting out a single line in the file Adafruit_EPD.h in the Adafruit_EPD library. At around line 29 add two slashes in front of this line:
#define USE_EXTERNAL_SRAM ///< use the external RAM chip on the EPD breakout
to make it a comment, effectively disabling the #define command:
//#define USE_EXTERNAL_SRAM ///< use the external RAM chip on the EPD breakout
Making these changes allowed me to run the demo program successfully.
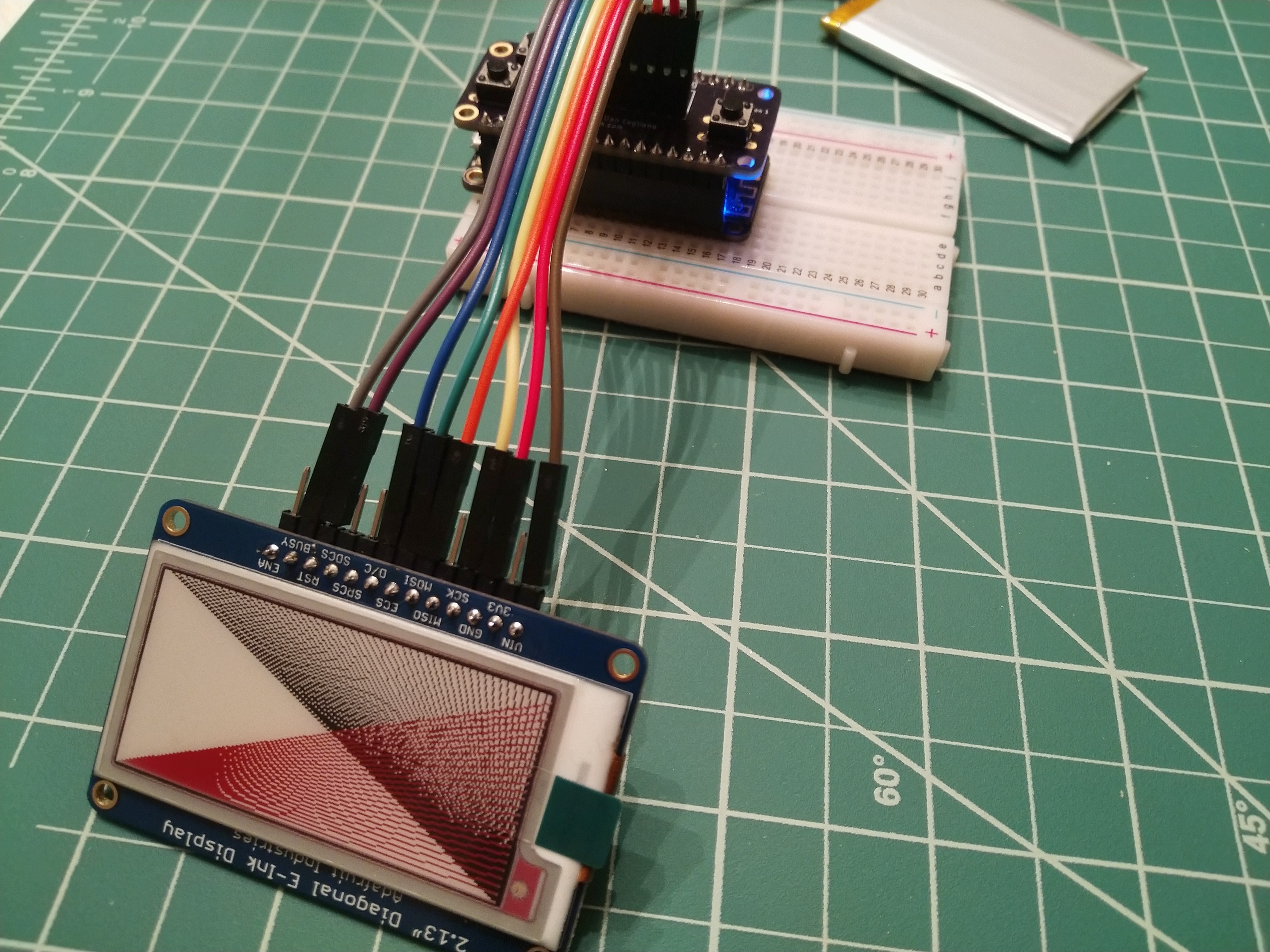
So far I have created a couple of sketches to work specifically with the Adafruit display, including a dynamic monthly calendar display and a random quote display using Adafruit’s quote API. I will be posting the code on GitHub for these and other sketches as I develop them for use with the e-Paper Featherwing. You may need to tweak the code to get it to work with different Adafruit display sizes.
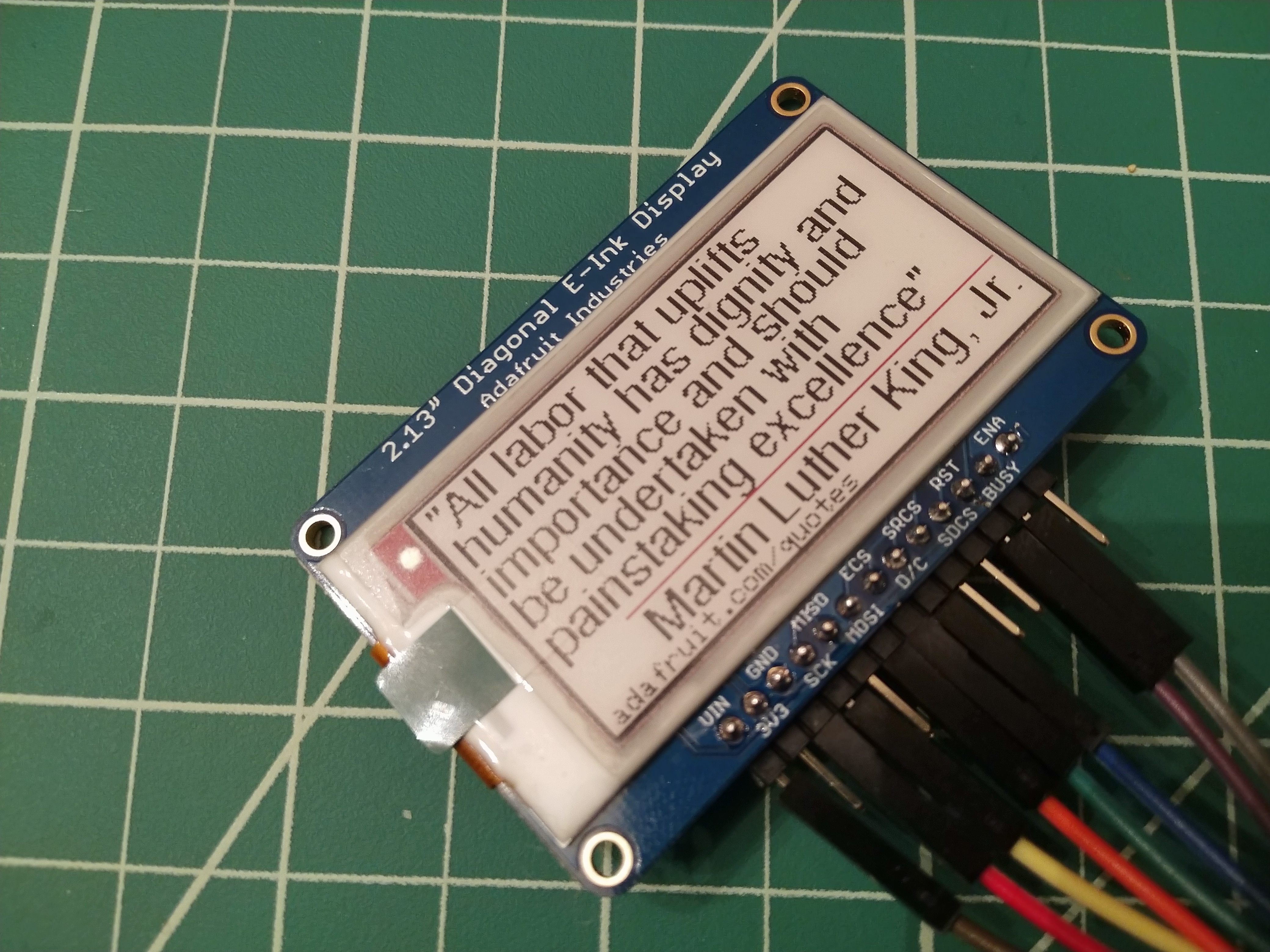